Collect Objects Block
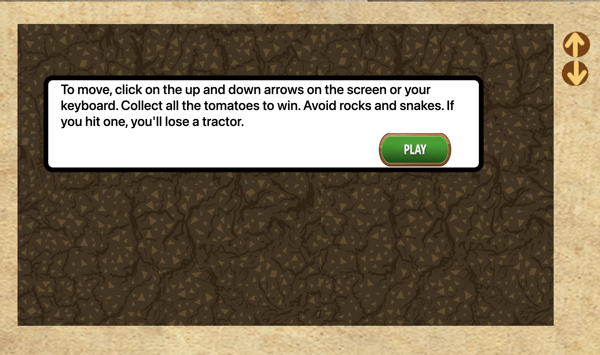
Using this Block
In the collect objects game, the player moves around a grid collecting “good” items and avoiding “bad” items. Unlike the Moving Targets block, where the items to be clicked move, in this game block, it’s the player that moves and the items are stationary.
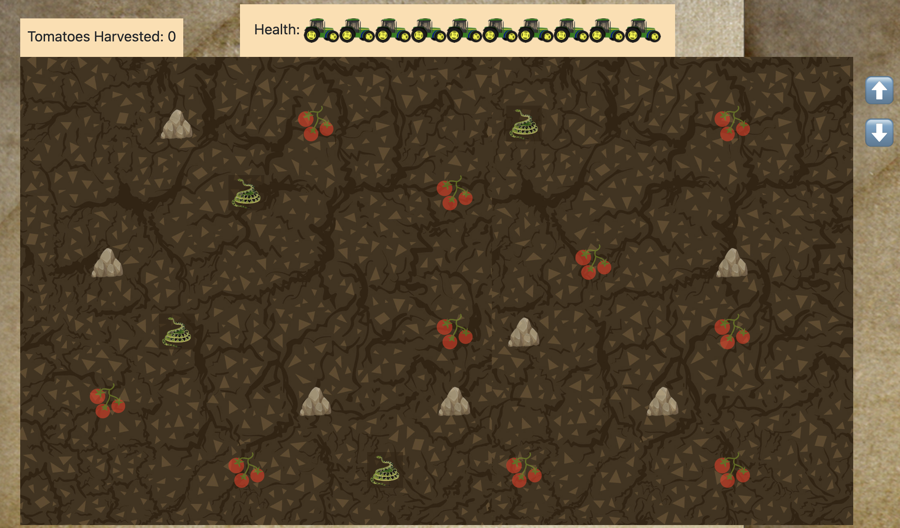
Example use
In a game about farming, the tractor moves through the field harvesting tomatoes and avoiding rocks and snakes.
Making it your own
What’s in the block
Like every block, the collect_objects block has two files in the folder:
- An HTML file: collect_objects.html . You should not have to change anything in this file.
- A config file: collect_objects_config.js – This is where you will make any changes to create your unique game.
As with most blocks, it also has an images folder. Any images used specifically in this block will be saved here. This will include the game background and the “targets”.
There is also an audio folder that has the sounds to play when you collect a good item or hit a bad item.
The config file
In the collect_objects_config.js file you’ll see two sections. As with all blocks, there is an options object. The options contain instructions on how to play the game and where to go after you have won the game.
Page options
const options = {
instructionsPage1: "<h3>To move, click on the up and down arrows on the screen or your keyboard. " +
"Collect all the tomatoes to win. Avoid rocks and snakes. If you hit one, you'll lose a tractor.</h3>" ,
backgroundImage: "images/harvest_game_background.png",
healthIcon: "images/tractor_small.png",
characterImage: "images/tractor.png",
maxHealthPoints: 10,
scoreLabel: "Tomatoes Harvested:",
completionURL: "../next_block/next_block.html",
successText: "<p>Great job! You harvested all the tomatoes!</p>",
loseText: "<p>Your tractor broke down!</p><p>You have to start over again with a new tractor!</p>",
goodSound: "audio/bell.wav", // Change if you want a different sound when they collect the object ,
badSound: "audio/wrong_buzzer_sound.mp3" // Change if you want a different sound when they hit an obstacle
};
- InstructionsPage1 can be either html or just plain text. For plain text, simply paste the instructions text you want between the quotation marks after the colon.
- backgroundImage – put the URL for the background image here, be sure it is in quotes.
- healthIcon – this image shows the number of lives. It should be small and obvious. In the example above, we have small tractors. If the game character is a person, it could be hearts or a small version of the character.
- characterImage – this image is the character going across the screen collecting objects. In our example, it’s a tractor but it could be a boat in an ocean, a person, animal or whatever your imagination dictates
- maxHealthPoints – this is the number of lives the player has. Make this number larger or smaller to make the game easier or harder.
- scoreLabel – What to label the score. In our case, it is tomatoes harvested. This increases every time the player collects a ‘reward’ item.
- completionURL – you’ll see this in every block. It is the URL where you want to go on the completion of this assessment.
- successText – text to show when player collects all the objects.
- loseText – text to show when player loses all health points.
- goodSound – audio that plays when player collects an object.
- badSound – audio that plays when player hits an obstacle.
Reward (Objects) and Obstacles
const displayItems = [
{
itemID: "tomato",
itemSymbol: "0",
itemImage: "images/tomato.png",
itemType: "reward"
},
{
itemID: "rock",
itemSymbol: "1",
itemImage: "images/rock.png",
itemType: "obstacle"
},
{
itemID: "snake",
itemSymbol: "2",
itemImage: "images/snake-gif.gif",
itemType: "obstacle"
},
];
DO NOT CHANGE THE itemSymbol VALUES!
NOTE: The images should have a transparent background. The image files names should be in quotes, as shown above, with a comma after the end quote.
The first item is the reward. Change the itemImage to the image file for your game. You can change the itemID to be a different name, however, if the itemID for your balloons or fish or whatever you are collecting is “tomato”, the game will work just as well. Just make sure each of the three item IDs is different.
The next two items are the obstacles. For each, give an image file and, if you want, change the name.
What if I don’t want two different types of obstacles?
Maybe you are catching corn borers in a corn field and the only type of obstacle you want is corn stalks. If you only want one type of obstacle, use the same image for the second and third items. Just be sure you give them different IDs like “corn” and “more_corn” .