Caves and Trees: Math
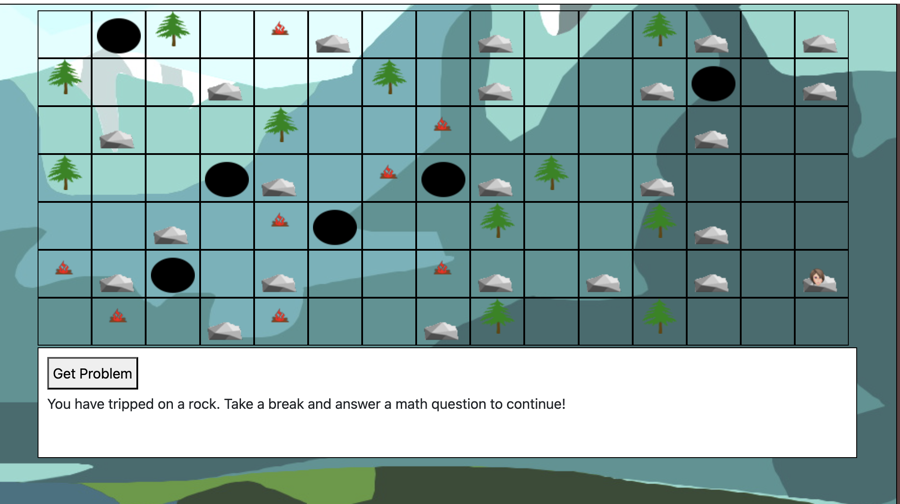
Using this Block
This is a combination game and assessment block. You might also consider it practice or instruction. All the things! The player rolls a die to advance through the game. Caves send the player back, trees can be climbed to the next row. If a player lands on one type of square, they must answer a problem to advance. A second type of square asks a harder problem but the player advances twice as far.
There is quite a bit in the config file , but the good news is that you can leave it exactly the way it is, if you like. In fact, if you aren’t very into coding or are in a hurry, you can probably just change the image and audio links, the next block link and maybe edit the difficulty of the problems at the end and have a completely new game.
Making it your own
What’s in the block
Like every block, the runner block has two files in the folder:
- An HTML file: caves_and_trees.html . You should not have to change anything in this file.
- A config file: caves_and_trees_config.js – This is where you will make any changes to create your unique game.
As with most blocks, it also has an images folder. Any images used specifically in this block will be saved here. This will include the game background, player character and the obstacles.
There is also an audio folder that has the sounds to play when you encounter an object.
The config file
In the caves_and_trees_config.js file you’ll see five sections. Pretty much everything in the config file can be left as is, but you have the ability to make changes.
Options object
As with all blocks, there is an options object. The options include instructions on how to play the game and where to go after you have won the game.
There are a few options here, and you can keep most of them the same if they suit you.
const options = {
title: "Caves and Trees",
background_image: "images/mountain_background.jpg",
win_image: "images/won_sledding.jpg", //OPTIONAl Use "" if no win image.
blank_image: "images/smalls/blank.png",
dice_roll_sound: "sounds/diceroll.mp3",
completionURL: "../next_block/next_block.html"
};
title – This is the title that will show up when they start the game. You can leave it as Caves and Trees or change it to fit your game.
background_image: The background image for your game. This will also show up as the background image on the title screen.
win_image: If you want a different image to show up when the player wins the caves and trees game, put the link here. Otherwise, just use “” and it will show the same background image.
blank_image: This is the image that shows up in squares where nothing happens, that is, they don’t go up or down or answer a question. The default is this image that is just a blank square. Personally, I’d just leave it as is.
dice_roll_sound: This is the sound of a dice rolling. Again, I’m not sure why you’d want to change it, but you can replace it with another sound if you like.
completionURL – you’ll see this in every block. It is the URL where you want to go on the completion of this game. This, you do want to change to your next block.
Characters Object
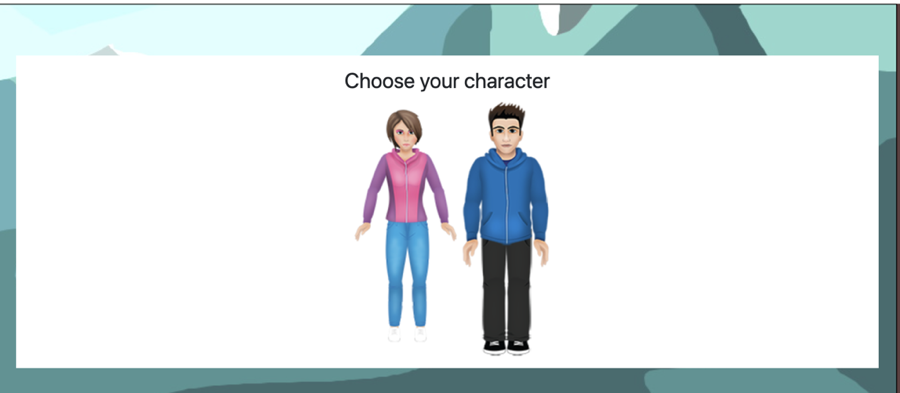
The characters object gives the images and alt text for the characters. You can leave as is to use the characters provided or use your own by change the images
const characters = [
{
id: "character1",
image: "images/angie_med.png",
alt: "girl",
piece_image: "images/smalls/blank_girl.png"
},
{
id: "character2",
image: "images/sam_med.png",
alt: "boy",
piece_image: "images/smalls/blank_boy.png"
},
];
id: Leave this. It just specifies an ID for selecting a character.
image: This is the image that will show up on the screen where players select a character (see above). You can just use the characters provided but , in a larger game where you have a narrative involving certain characters, you might want to include them here.
alt: This is the alt text for the image.
piece_image: This is a much smaller image that will show up on the game board as the character you chose. Again, you can just use the characters provided if you want.
Squares Object
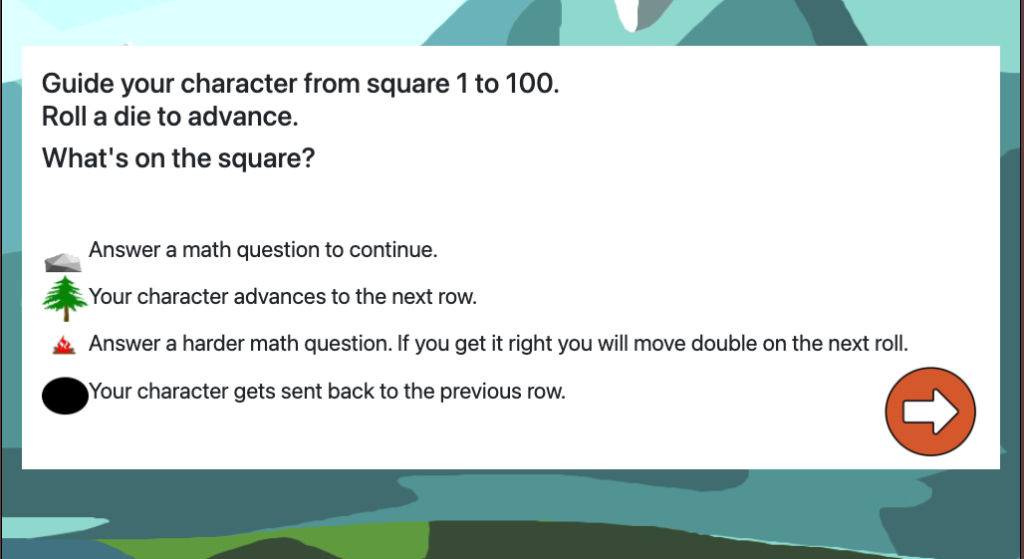
There are five types of squares: do nothing, math problem, go up a row, harder math problem, drop down a row.
const squares = [
{
id: "blank",
// instruction_text: There is no instruction text for a blank square
landing_text: "You have landed on nothing. Roll again!",
image: "images/smalls/blank.png",
alt: "Empty square",
square_type: 0, // obstacle 0 (do nothing)
sound: "sounds/diceroll.mp3",
action: "roll"
},
{
id: "rock",
instruction_text: "Answer a math question to continue.",
landing_text: "You have tripped on a rock. Take a break and answer a math question to continue!",
image: "images/smalls/rock2.png",
alt: "rock",
square_type: 1, // obstacle 1 (do a problem to proceed),
action: "problem-1"
},
{
id: "tree",
instruction_text: "Your character advances to the next row.",
landing_text: "You have landed on a tree. You get to climb up to the next row!",
image: "images/smalls/tree.png",
alt: "tree",
square_type: 2, // tree (go up one row)
sound: "sounds/go_up.mp3",
action: "move-up"
},
{
id: "fire",
instruction_text: "Answer a harder math question. If you get it right you will move double on the next roll.",
landing_text: "You have landed on a campsite. Answer a harder math question to advance double on the next roll!",
image: "images/smalls/fire.png",
alt: "camp fire",
square_type: 3, // obstacle 2 (do a harder problem to proceed)
sound: "sounds/fire.mp3",
action: "problem-2"
},
{
id: "cave",
instruction_text: "Your character gets sent back to the previous row.",
landing_text: "You fell in a cave. Sorry, you fell back down to the previous row!",
image: "images/smalls/hole.png",
alt: "black hole",
square_type: 4, // hole (go down one row)
sound: "sounds/falling.mp3",
action: "move-back"
},
];
The good news here is that each square has the exact same fields.
HINT: You probably want to leave the first square, ‘blank’ as is. That will just be an empty square.
id: unique name for this type of square. You can change this or leave it as suits you but it needs to be a valid ID, that is no spaces, can include letters, numbers, _ or $ but cannot start with a number.
instruction_text – the text that will show on the instruction page. (See above for example)
landing text – This is the text shown below the screen when a player lands on this type of square (see below for example).
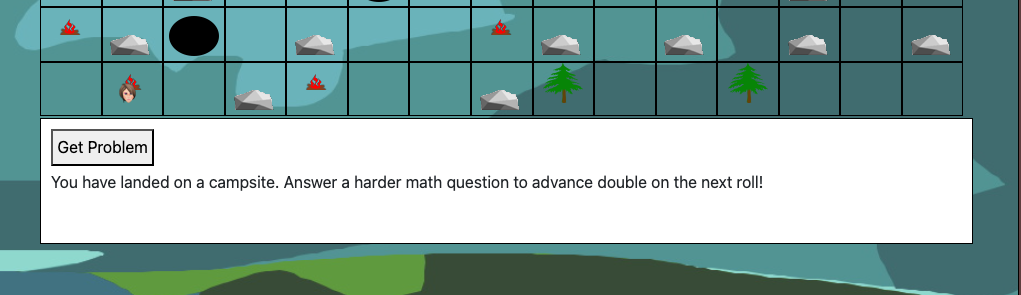
image – The link to the image for that type of square. If you like trees, caves, rocks and fires, you can just leave these or you can swap in your own.
alt text – The alt text for the image
square_type: The type of square (0=blank, 1=math problem , 2= go up a row, 3= harder problem, 4= go down a row). These numbers will match up to the map below.
sound – The link to the audio file that plays when they land on that square. If you like the sounds we have, you can just leave these, or you can swap your own.
action – What action to take on this square. You should probably just leave this. The action is either “roll”, which means after this square they will roll again, “problem-1”, which is an easier math problem, “move-up” which means to go up a row, “problem-2” , which is a harder math problem or “move-back” which means to go down a row.
Maps Object
You can just leave this as is, but you can change it if you want. As the comments say, each number matches up with a square type that is defined above in the squares object. The game starts in the bottom left square and ends at the top right (see below). A 0 will have a blank square, 1 will be an obstacle (up above I made this an image of a rock, but you can change it if you want. To change the map, just change the numbers. For example, to have a cave in the second square, just change the number in that square from 0 to 4.
// Square types
// 0: blank (nothing on this square)
// 1: obstacle 1 (do a problem to proceed)
// 2: tree (go up one row)
// 3: obstacle 2 (do a harder problem to proceed)
// 4: cave (go down one row)
const map = [
[0, 4, 2, 0, 3, 1, 0, 0, 1, 0, 0, 2, 1, 0, 1],
[2, 0, 0, 1, 0, 0, 2, 0, 1, 0, 0, 1, 4, 0, 1],
[0, 1, 0, 5, 2, 0, 0, 3, 0, 0, 0, 0, 1, 0, 0],
[2, 0, 0, 4, 1, 0, 3, 4, 1, 2, 0, 1, 0, 0, 0],
[0, 0, 1, 0, 3, 4, 0, 0, 2, 0, 0, 2, 1, 0, 0],
[3, 1, 4, 0, 1, 0, 0, 3, 1, 0, 1, 0, 1, 0, 1],
[0, 3, 0, 1, 3, 0, 0, 1, 2, 0, 0, 2, 0, 0, 0]
];
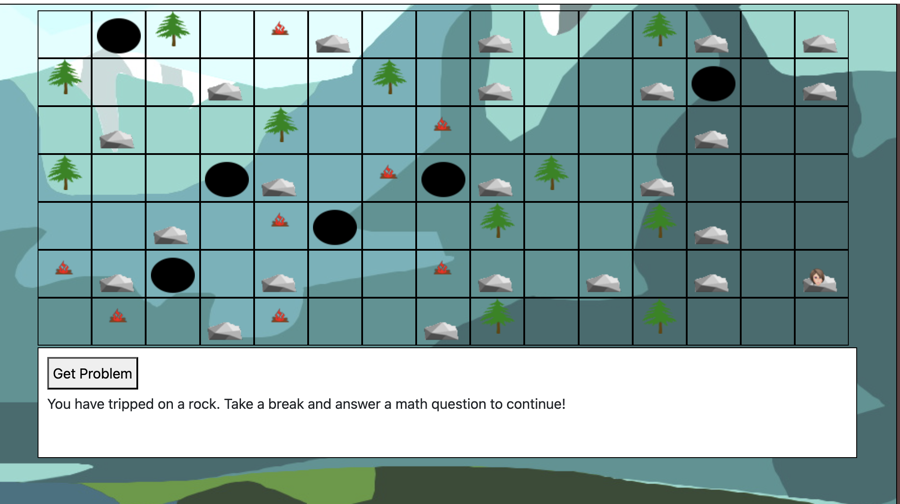
Math Problems Object
/*
OPERATOR CAN BE / FOR DIVIDE , * FOR MULTIPLY, + FOR ADDITION OR - FOR SUBTRACTION
*/
const mathProblems = {
easy: {operator:"*", minValue1:4, maxValue1: 400, minValue2:2, maxValue2:20},
hard: {operator:"/", minValue1:16, maxValue1: 2500, minValue2:4, maxValue2:50},
}
operator: for division, multiplication, addition or subtraction, select from one of these four operators / * + –
You can mix operators. For example, the easy problems could be multiplying numbers of up to 400, while the division problems could be dividing problems with divisors (the number to be divided) up to 2,500.
minValue1 and maxValue1: Give the minimum value and maximum value for the first number in the problem.
minValue2 and maxValue2: Give the minimum value and maximum value for the second number in the problem.
NOTES!
While the order of the numbers for minimum and maximum values doesn’t matter for addition or multiplication, for subtraction and division, order DOES matter.
SUBTRACTION: For the first value, in the case of subtraction this would be the number you are subtracting FROM (if you didn’t know, this is called the minuend) and the second number would be the number you are subtracting (this is the subtrahend).
DIVISION: For the first value, enter minimum and maximum values for the number you are dividing (this is called the dividend, in case you were wondering). The minimum and maximum values for the second value are for the number you are dividing by (called the divisor).